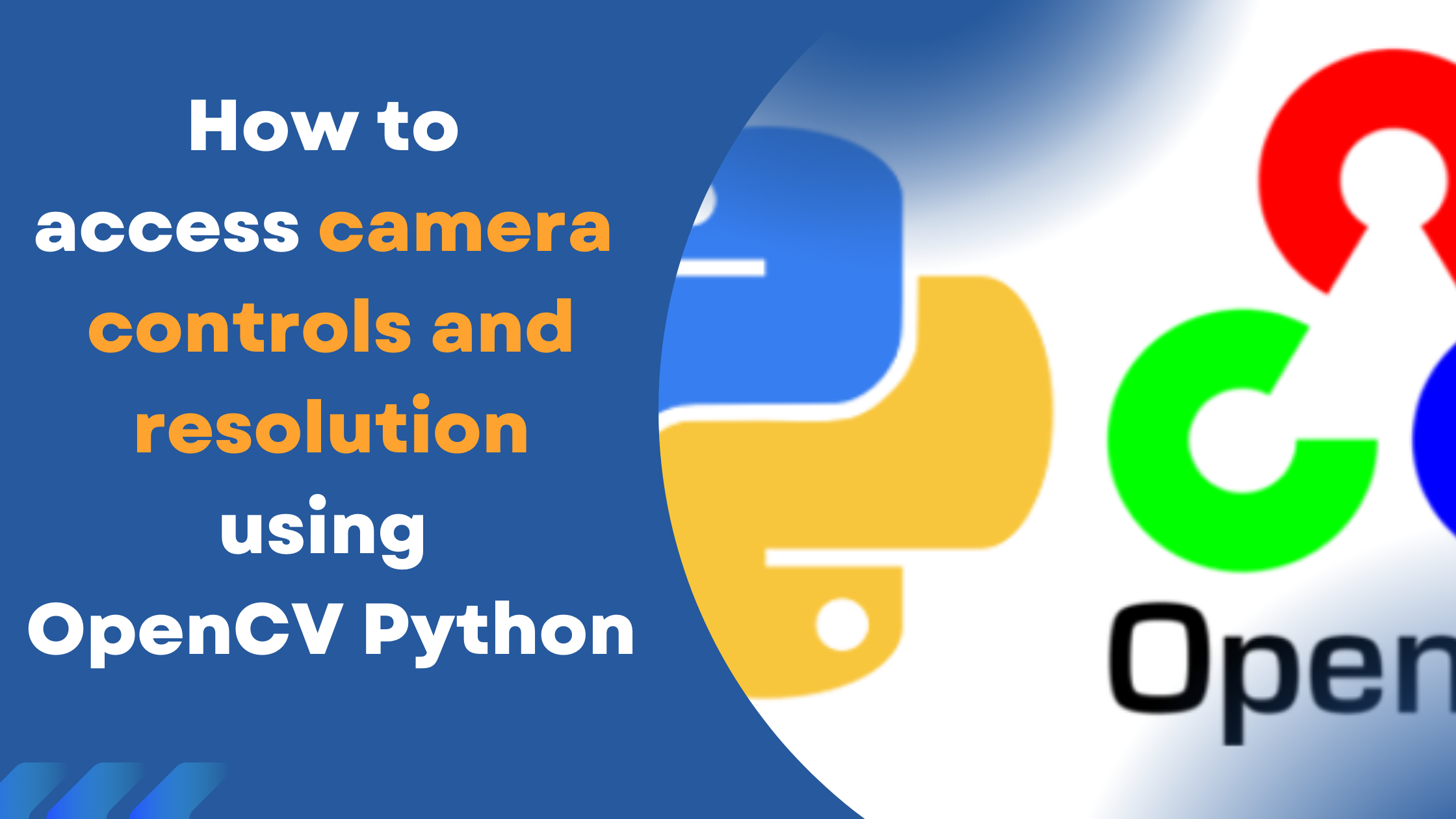
How to access camera controls and resolution using OpenCV Python
The default camera settings will not be suitable for your needs. The majority of cameras has both an auto and manual mode for capturing good pictures. The ability to tune camera parameters according to your lighting environment and use case is a key skill in imaging, especially when using OpenCV Python. (click here to know How to access the USB camera using OpenCV python )
In this article, we’ll show you how to use OpenCV Python to access basic camera controls like brightness and contrast, as well as change image formats and resolutions.
import cv2
FRAME_WIDTH=3
FRAME_HEIGHT=4
FRAME_RATE=5
BRIGHTNESS=10
CONTRAST=11
SATURATION=12
HUE=13
GAIN=14
EXPOSURE=15
#Opens the first imaging device
cap = cv2.VideoCapture(0)
#Check whether user selected camera is opened successfully.
if not (cap.isOpened()):
print("Could not open video device")
#To set the resolution
cap.set(cv2.CAP_PROP_FOURCC, cv2.VideoWriter_fourcc('M', 'J', 'P', 'G'))
#cap.set(cv2.CAP_PROP_FOURCC, cv2.VideoWriter_fourcc('U', 'Y', 'V', 'Y'))
cap.set(cv2.CAP_PROP_FRAME_WIDTH, 640)
cap.set(cv2.CAP_PROP_FRAME_HEIGHT, 480)
print("Width = ",cap.get(cv2.CAP_PROP_FRAME_WIDTH))
print("Height = ",cap.get(cv2.CAP_PROP_FRAME_HEIGHT))
print("Framerate = ",cap.get(cv2.CAP_PROP_FPS))
print("Format = ",cap.get(cv2.CAP_PROP_FORMAT))
Brightness=cap.get(cv2.CAP_PROP_BRIGHTNESS)
Contrast=cap.get(cv2.CAP_PROP_CONTRAST)
Saturation=cap.get(cv2.CAP_PROP_SATURATION)
Gain=cap.get(cv2.CAP_PROP_GAIN)
Hue=cap.get(cv2.CAP_PROP_HUE)
Exposure=cap.get(cv2.CAP_PROP_EXPOSURE)
while(True):
# Capture frame-by-frame
ret, frame = cap.read()
# Display the resulting frame
cv2.imshow('preview',frame)
#Waits for a user input to quit the application
k = cv2.waitKey(1)
if (k == 27):#Esc key to quite the application
break
elif k == ord('g'):
print("******************************")
print("Width = ",cap.get(FRAME_WIDTH))
print("Height = ",cap.get(FRAME_HEIGHT))
print("Framerate = ",cap.get(FRAME_RATE))
print("Brightness = ",cap.get(BRIGHTNESS))
print("Contrast = ",cap.get(CONTRAST))
print("Saturation = ",cap.get(SATURATION))
print("Gain = ",cap.get(GAIN))
print("Hue = ",cap.get(HUE))
print("Exposure = ",cap.get(EXPOSURE))
print("******************************")
elif k == ord('w'):
if(Brightness <= 0):
Brightness = 0
else:
Brightness-=1
cap.set(BRIGHTNESS,Brightness)
print(Brightness)
elif k == ord('s'):
if(Brightness >= 255):
Brightness = 255
else:
Brightness+=1
cap.set(BRIGHTNESS,Brightness)
print(Brightness)
# When everything done, release the capture
cap.release()
cv2.destroyAllWindows()
Supported UVC controls:
Brightness: #10. CV_CAP_PROP_BRIGHTNESS Brightness of the image (only for cameras).
Contrast: #11. CV_CAP_PROP_CONTRAST Contrast of the image (only for cameras).
Saturation: #12. CV_CAP_PROP_SATURATION Saturation of the image (only for cameras).
Hue: #13. CV_CAP_PROP_HUE Hue of the image (only for cameras).
Gain: #14. CV_CAP_PROP_GAIN Gain of the image (only for cameras).
Manual Exposure: #15. CV_CAP_PROP_EXPOSURE Exposure (only for cameras).
Not Supported UVC Controls:
Auto white balance
Auto Exposure
Manual white balance
Gamma correction
Sharpness