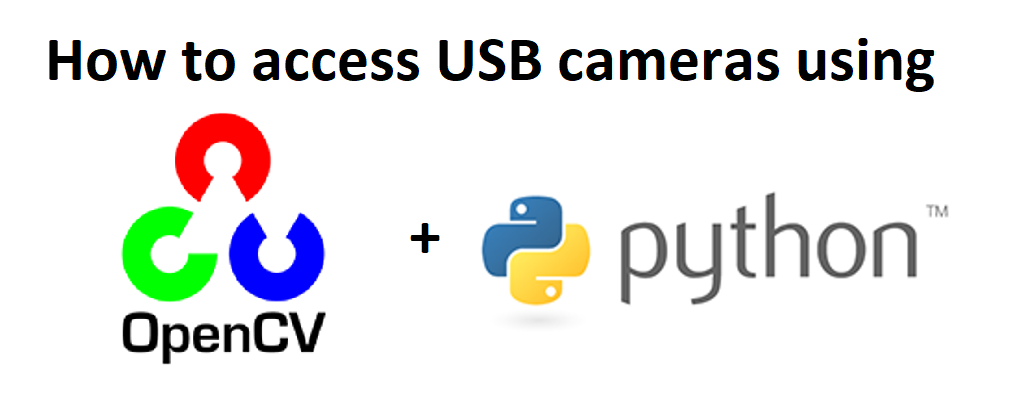
How to access the USB camera using OpenCV python in windows
A Quick Overview of OpenCV:
OpenCV (Open-Source Computer Vision Library) is a free software library for computer vision, machine learning and image processing. OpenCV was created to provide a common platform for computer vision applications and to help commercial products integrate machine perception more quickly. Since OpenCV is a BSD-licensed product, it is simple for businesses to use and modify the code.
Open Computer Vision (OpenCV) image processing bundle that includes functions for basic image decoding, enhancement, color space conversion, object recognition, and object tracking, among other things. Internally, OpenCV uses the DirectShow (Windows) and V4L2 (Linux) frameworks to access frames from the camera. As algorithm developers, we are focused on improving our results.
The ultimate aim for this blog is to explain how to use a simple Python script to access and use USB cameras in OpenCV.
USB Cameras:
USB cameras are UVC-compliant and support Plug & Play on Windows/Linux, eliminating the need to manually install additional device drivers. This camera will work with the native UVC drivers for Windows and Linux operating systems. To access the camera and demonstrate some of its features, use any DirectShow (for Windows) or V4L2 (for Linux) based application.
We installed OpenCV and Python on a Windows 10 computer and tried the laptop webcam streaming.
Download the Python:
Step 1: Use the link to download the python : https://www.python.org/downloads/release/python-382/
Step 2: Go to the above URL and scroll down to the bottom of the page to find the executable file. Please take a look at the image below.

Step 3: Once we have python installed, we can check the version by running the below command
C:\Users\mark>python –version
Python 3.8.2
Step 4: open the command prompt window and install the OpenCV by using below pip command
C:\Users\mark>pip install opencv-python
Open Python IDLE and type following codes in Python terminal.
>>> import cv2
>>> print cv2__version__
If the results are printed out without any errors, and print the OpenCV version which you have installed congratulations !!! You have installed OpenCV-Python successfully.
Step 5: Copy the below sample code and save it as webcam.py
import cv2
#Capture video from webcam
cap = cv2.VideoCapture(0)
while(True):
# Capture each frame of webcam video
ret,frame = cap.read()
cv2.imshow("My cam video", frame)
# Close and break the loop after pressing "x" key
if cv2.waitKey(1) &0XFF == ord('x'):
break
# close the already opened camera
cap.release()
# close the window and de-allocate any associated memory usage
cv2.destroyAllWindows()
Step 6:
Open the window power shell application and Run the application by using the below command
C:\python\python38> python .\webcam.py
Output:

Hope this will be useful for you to access images from your USB camera using OpenCV python in windows.