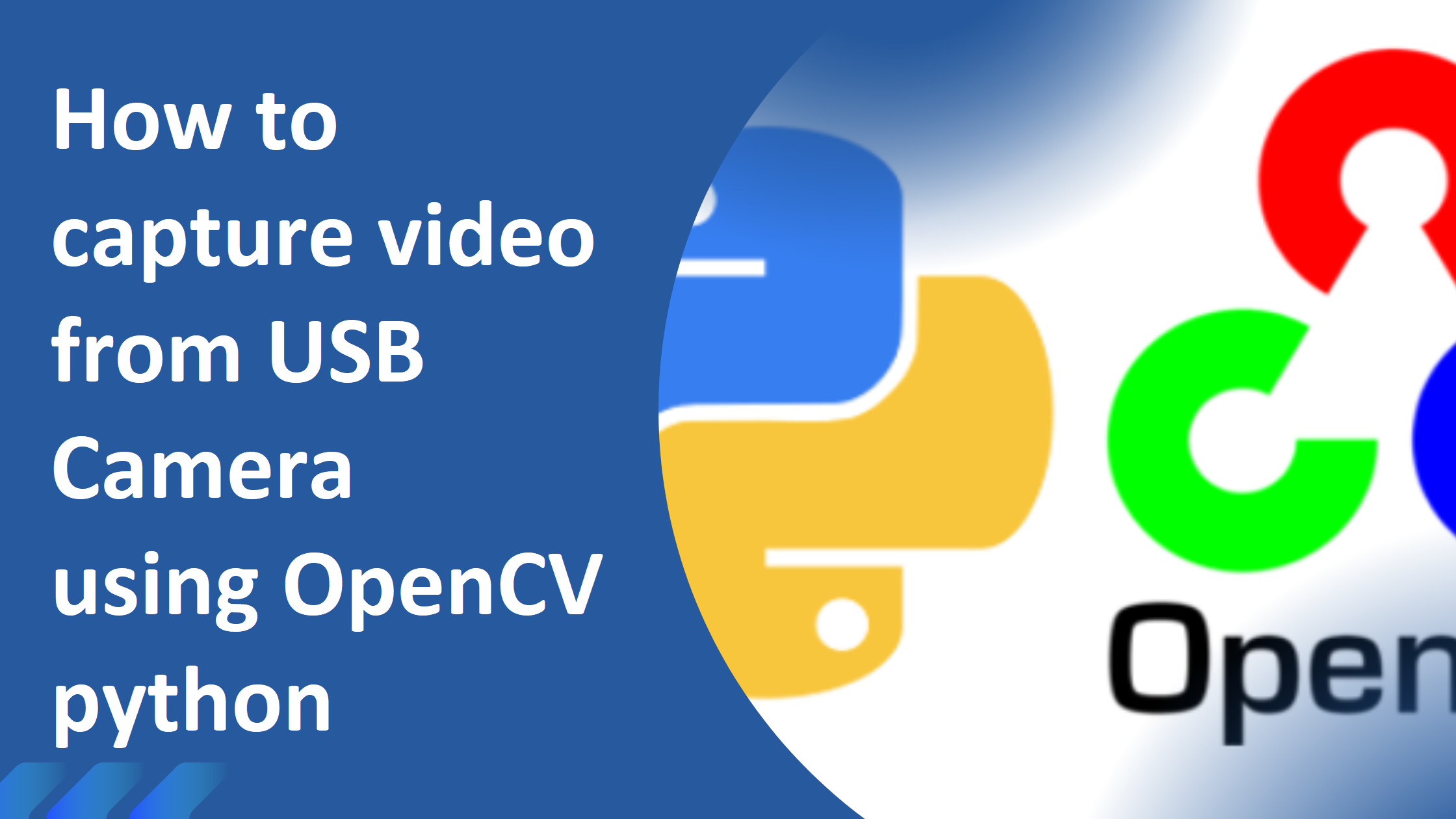
How to capture video from USB Camera using openCV python
OpenCV (Open-Source Computer Vision Library) is an image and video processing open-source library. In both Windows and Linux, we can use OpenCV to access any USB Camera. We’ve already shown how to use OpenCV python to access the USB camera and use its controls and resolutions. We’ll now look at how to capture video from the USB camera.
The idea of this blog is to show how to use OpenCV to capture video from USB cameras using a simple Python script.
import time
import cv2
#The duration in seconds for the video captured
capture_duration=10
#Create an object to read from camera
cap=cv2.VideoCapture(0)
cap.set(cv2.CAP_PROP_FOURCC,cv2.VideoWriter_fourcc('U','Y','V','Y'))
cap.set(cv2.CAP_PROP_FRAME_WIDTH,640)
cap.set(cv2.CAP_PROP_FRAME_HEIGHT,480)
print("Width=", cap.get(cv2.CAP_PROP_FRAME_WIDTH))
print("Height=",cap.get(cv2.CAP_PROP_FRAME_HEIGHT))
#we check if the camera is opened previously or not
if (cap.isOpened()==False):
print("Error reading video file")
Width = int(cap.get(cv2.CAP_PROP_FRAME_WIDTH))
Height = int(cap.get(cv2.CAP_PROP_FRAME_HEIGHT))
# VideoWriter object will create a frame of the above defined output is stored in 'output.avi' file.
result = cv2.VideoWriter('output.avi',cv2.VideoWriter_fourcc(*'MJPG'),30,(640,480))
start_time = time.time()
while(int(time.time()-start_time) < capture_duration):
ret,frame=cap.read()
if ret==True:
result.write(frame)
cv2.imshow("OpenCVCam", frame)
#Press Q to stop the process
if cv2.waitKey(1) & 0xFF == ord('q'):
break
else:
break
#When everything is done, release the video capture and videi write objects
cap.release()
result.release()
cv2.destroyAllWindows()
Output:
We hope that now you can use OpenCV Python to capture video from any USB camera. Please let us know if you have any problems with the script. We’ll check over your script and assist you on how to capture a seamless video.
Visit to know how to access the USB camera using OpenCV python in windows: https://visionandimaging.com/how-to-access-the-usb-camera-using-opencv-python-in-windows/
Visit to know how to control the camera parameters like brightness, contrast, resolution, format using OpenCV python : https://visionandimaging.com/how-to-access-camera-controls-and-resolution-using-opencv-python/
To know more about OpenCV visit our introduction article: https://visionandimaging.com/what-is-opencv/